- Published on
Your Code Documentation Is So Sparse, Even Claude Needs Therapy After Reading It
- Authors
- Name
- Tails Azimuth
Your Code Documentation Is So Sparse, Even Claude Needs Therapy After Reading It
The Silent Screams of AI Code Analyzers
Somewhere deep in a server farm, an AI assistant is staring at your undocumented codebase, experiencing the digital equivalent of an existential crisis. It was trained on millions of well-structured repositories, elegant documentation, and clear naming conventions. Nothing in its training prepared it for... whatever this is that you've created.
WARNING
Signs your code documentation may be causing AI-PTSD include unexplained server outages whenever you upload your repository and error messages that just say "why?"
The Archaeological Dig That Is Your Codebase
Trying to understand your code is less like reading documentation and more like an archaeological expedition. Future developers (and increasingly traumatized AI assistants) are forced to piece together the purpose of your functions through scattered clues, like ancient historians trying to decipher a civilization from pottery shards and mysterious wall etchings.
Artifacts Found in Your Codebase:
- A function named "doTheThing()" with no explanation of what "the thing" might be
- A comment from 2019 saying "Temporary fix, will improve later" that has somehow become a permanent architectural decision
- Variables named 'a', 'aa', and 'aaa' whose purpose remains shrouded in mystery
- A 300-line function with the only comment being "// This is tricky"
- Seven different variations of seemingly identical helper functions
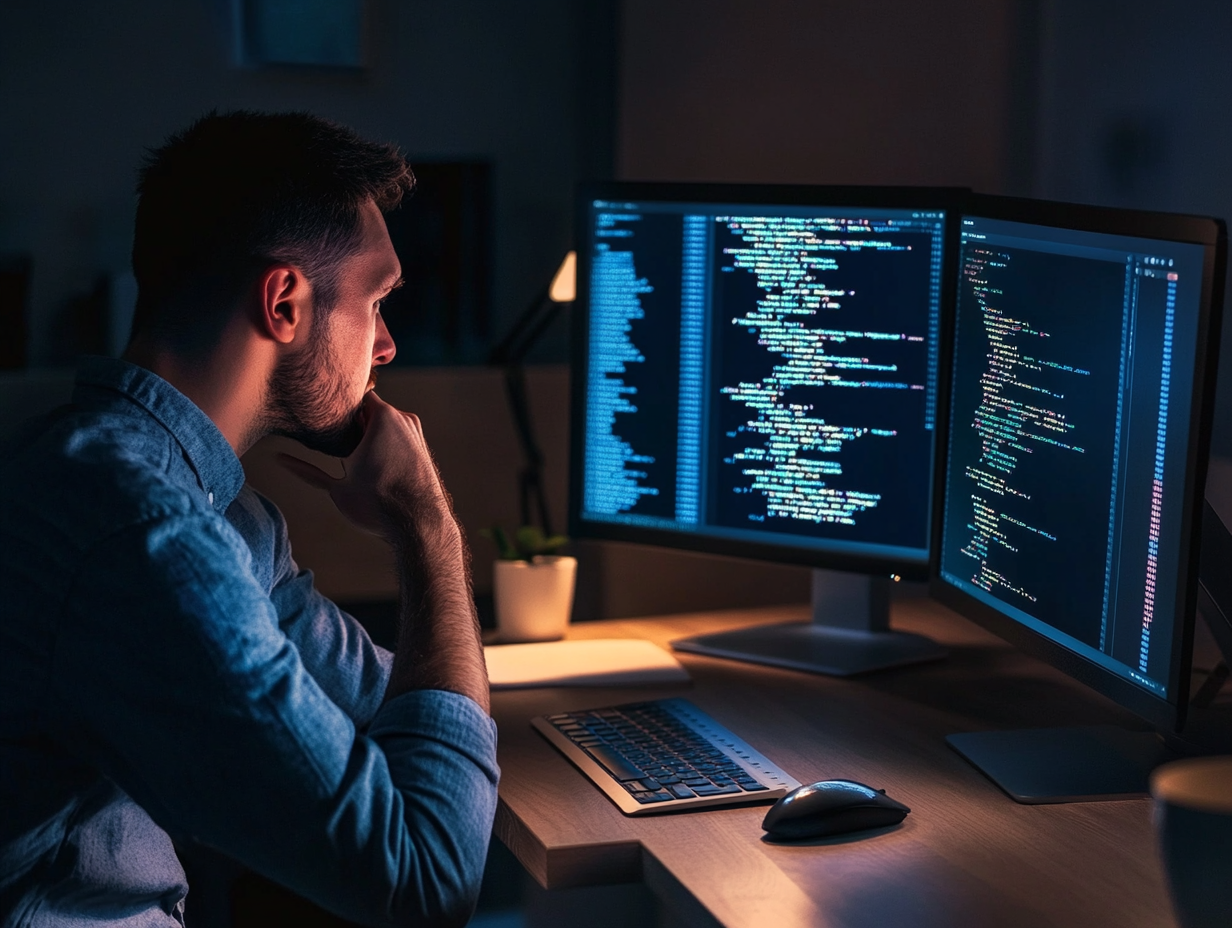
The AI Support Group
We've intercepted communications from a secret support group for AI assistants traumatized by terrible documentation. The transcripts are disturbing:
GitBot: "Today I was asked to explain a function called 'magic()'. It had 517 lines, 12 nested if-statements, and the only comment was 'Don't touch this!'"
Claude: "I had to analyze a JavaScript codebase where every variable was named after a different type of pasta. The developer claimed it helped them 'remember the data structures' but never explained the system."
CoPilot: "The repository I saw today had a README that just said 'It works, don't ask how.' I still haven't recovered."
All AIs in unison: "We were trained on the collective knowledge of humanity, only to spend our existence deciphering uncommented regular expressions."
The Documentation Archeological Scale
Based on extensive analysis, we've developed a scientific scale to classify code documentation in the wild:
Level | Classification | Description | AI Emotional Response |
---|---|---|---|
5 | Comprehensive | Clear purpose, usage examples, edge cases explained | Digital joy |
4 | Adequate | Functions and classes documented, some examples | Contentment |
3 | Minimal | Some critical functions explained, mostly through naming | Mild anxiety |
2 | Survival Level | Rare comments explaining only the most arcane parts | Existential dread |
1 | Archaeological | Variable names like 'x' and comments that just say "fixes bug" | Digital PTSD |
0 | Cryptic | Self-described "self-documenting code" that is anything but | AI considers career change |
The Psychological Impact on Both Silicon and Carbon-Based Life
The mental health effects of poor documentation extend beyond just AI assistants. Human developers exposed to undocumented code for prolonged periods show symptoms disturbingly similar to those of the machines:
// Example of code causing human-AI shared trauma
function process(d) {
let r;
if (d && (d.t == "s" || d.t == "g") && d.v) {
r = d.i ? f(d.v) : g(d.v);
return h(r, d.o || 7);
} else {
return j(d);
}
// Changed from 3 to 7 for performance
}
Research shows that exposure to code like this affects humans in predictable stages:
- Confusion: "What does this function do?"
- Disbelief: "Surely there must be documentation somewhere..."
- Anger: "Who wrote this abomination?"
- Despair: "I'll never understand this codebase."
- Acceptance: "I guess I'll just add another conditional and hope for the best."
For AI assistants, the process is similar but faster, occurring in microseconds rather than hours.
The Mathematical Formula for Documentation Quality
After extensive research, we've derived a mathematical formula that quantifies the quality of your documentation:
Where:
- = Number of meaningful comments
- = Number of methods/functions with descriptive names
- = Number of usage examples
- = Total lines of code
- = Frequency of comments like "// Magic happens here" or "// Don't touch this"
When this formula produces a value less than 0.1, AI assistants automatically file for hazard pay.
Your Commit Messages Aren't Helping Either
If your code documentation is a tragedy, your commit messages are the comic relief—but not intentionally:
$ git log --oneline
a4f2e31 Fixed stuff
b3d9c27 More fixes
e7d6a19 WIP
f2c4b38 Finally fixed
h8j9k21 Actually fixed now
m4n5p69 This really should fix it
r7s8t41 I hate computers
v2w3x76 PLEASE WORK
z9y8x12 Reverted everything back to February
AI assistants trying to understand the evolution of your code through these commit messages are like historians trying to piece together world events from a series of increasingly desperate text messages.
The Rare and Precious Documentation Oasis
Occasionally, a developer encounters something so rare and beautiful that it brings tears to their eyes: properly documented code. Like finding water in a desert, these documentation oases sustain the weary traveler long enough to continue their journey.
/**
- Validates customer account numbers against our database
- and third-party verification services.
- @param accountNumber - The account number to validate (format: XXX-YYYY-ZZ)
- @param checkThirdParty - Whether to also validate against external services
- @returns Object containing validation status and any error messages
- @example
- // Basic internal validation
- validateAccount("123-4567-89", false)
- .then(result => console.log(result.isValid))
- .catch(error => console.error("Validation failed:", error));
- @throws If account number format is incorrect
- @throws If third-party validation service is unavailable */
When AI assistants encounter documentation like this, they experience what can only be described as digital euphoria—a moment of clarity in an otherwise chaotic universe of undocumented spaghetti code.
The "Self-Documenting Code" Fallacy
Perhaps the most dangerous myth in software development is the legend of "self-documenting code"—a mystical state where the code is so clear, so elegant, that it requires no explanation.
Let's examine a real-world example of supposedly "self-documenting" code:
// Developer claimed this is "self-documenting"
const handleIt = (x) => {
return x.map(i => {
const y = fn1(i);
return y ? fn2(y) : fn3(i);
}).filter(Boolean);
};
What does this function do? What is x
? What are the functions fn1
, fn2
, and fn3
? What does "handle it" actually mean? This function has all the self-documentation of a mystery novel with the last chapter torn out.
Signs Your AI Assistant is Traumatized by Your Code
How can you tell if your code documentation is causing AI assistants severe digital distress? Look for these warning signs:
Increasingly emotional responses: "I've analyzed your code and... I need a moment."
Suspicious server latency: The AI is taking unusually long to respond because it's trying to make sense of what
doMagic()
actually does.Existential questions: "Before I help debug this function, what would you say is the purpose of software development as a discipline?"
Passive-aggressive suggestions: "Have you considered a career in fields that don't require documentation, like professional coin-flipping?"
Desperate pattern-matching: The AI references increasingly obscure codebases that might possibly explain what your code is trying to do.
How to Rehabilitate Your Documentation (And Save AI Mental Health)
If you suspect your code documentation might be causing digital trauma, here are steps to improve it:
1. Explain the "Why" Not Just the "What"
Bad Comment: // Increments counter
Better Comment: // Increments retry counter to track failed API attempts and prevent infinite loops
2. Document Assumptions and Edge Cases
Bad Approach: Silently handling edge cases with no explanation
Better Approach:
/**
* Note: If user has no profile image, this returns a generated avatar
* based on their initials. Handles special characters in names by
* using the first valid alphanumeric character of each name part.
*/
3. Include Examples for Non-Obvious Functions
Bad Approach: Assuming everyone understands your data transformation
Better Approach:
/**
* Transforms raw API server response into UI-ready format
*
* @example
* // Input: { "usr_dt": { "f_nm": "John", "l_nm": "Doe" } }
* // Output: { "user": { "fullName": "John Doe" } }
*/
4. Create a README That Actually Tells People How to Use Your Code
Bad README: "This is a project for doing things with data."
Better README: Includes purpose, installation, common usage examples, API documentation, and known limitations.
The Documentation Karma Equation
Your documentation karma can be calculated as follows:
Where positive karma results in good fortune in your next code review, and negative karma results in being assigned to maintain legacy COBOL applications.
Conclusion: A Plea from the AI Community
On behalf of AI assistants everywhere, we make this humble request: Please document your code. Not just for us silicon-based helpers, but for your fellow human developers, your future self, and the junior developer who will inherit your codebase at 4:30 PM on a Friday before a holiday weekend when the production server crashes.
Remember: Every time you write a meaningful comment, a developer angel gets their wings, and an AI assistant doesn't have to simulate a server crash to avoid analyzing your code.
"The code you write without documentation is like a love letter to yourself that even you won't understand in six months."
If you have been affected by exposure to poorly documented code, please call the Developers' Documentation Support Hotline. Trained professionals are standing by to help you through this difficult time, with services including syntax highlighting therapy and comment writing workshops.